Firebase from Google offers a variety of mobile services. In a previous post, I demonstrated how to use Firebase for app distribution. In this post I’ll show you how you can implement push notifications in your .NET MAUI app using Firebase. We’ll do this using the NuGet package Plugin.Firebase, which was just updated to support .NET MAUI. The GitHub repository includes a lot of good information, so please visit it for more documentation.
Note! This plugin only supports Android and iOS. Therefore this will not work if you’re planning on targeting Mac Catalyst and/or Windows.
Setup in Firebase Console
First we’ll have to setup our project in the Firebase Console. Check out the GitHub documentation for the plugin here to see how that’s done. Make sure that when you register the bundle ID / package name in Firebase that it matches the one set in your .NET MAUI project (ApplicationId
in your .csproj file).
Create your .NET MAUI project
Start off by creating your .NET MAUI project in Visual Studio and install the NuGet package Plugin.Firebase.
If you’re on Windows, you might encounter an error when trying to install via the NuGet Package Manager. If that happens, try installing the package via the dotnet CLI instead:
dotnet add package Plugin.Firebase
Since the plugin doesn’t support Mac Catalyst or Windows, we’ll have to remove these as target frameworks from our .csproj:
<TargetFrameworks>net6.0-android;net6.0-ios;net6.0-maccatalyst</TargetFrameworks>
<TargetFrameworks Condition="$([MSBuild]::IsOSPlatform('windows'))">$(TargetFrameworks);net6.0-windows10.0.19041.0</TargetFrameworks>
Make sure to also remove them from SupportedOSPlatformVersion
and TargetPlatformMinVersion
:
<SupportedOSPlatformVersion Condition="$([MSBuild]::GetTargetPlatformIdentifier('$(TargetFramework)')) == 'ios'">14.2</SupportedOSPlatformVersion>
<SupportedOSPlatformVersion Condition="$([MSBuild]::GetTargetPlatformIdentifier('$(TargetFramework)')) == 'maccatalyst'">14.0</SupportedOSPlatformVersion>
<SupportedOSPlatformVersion Condition="$([MSBuild]::GetTargetPlatformIdentifier('$(TargetFramework)')) == 'android'">21.0</SupportedOSPlatformVersion>
<SupportedOSPlatformVersion Condition="$([MSBuild]::GetTargetPlatformIdentifier('$(TargetFramework)')) == 'windows'">10.0.17763.0</SupportedOSPlatformVersion>
<TargetPlatformMinVersion Condition="$([MSBuild]::GetTargetPlatformIdentifier('$(TargetFramework)')) == 'windows'">10.0.17763.0</TargetPlatformMinVersion>
<SupportedOSPlatformVersion Condition="$([MSBuild]::GetTargetPlatformIdentifier('$(TargetFramework)')) == 'tizen'">6.5</SupportedOSPlatformVersion>
Once that’s done, refer to the updated documentation for the NuGet package on how to make it work with .NET MAUI. Add the GoogleService-Info.plist
and google-services.json
files as described and setup the initialization in your MauiProgram.cs
. An important note here: add these preprocessor using statements in your MauiProgram.cs
to make sure the correct platform implementation gets loaded:
...
#if IOS
using Plugin.Firebase.iOS;
#else
using Plugin.Firebase.Android;
#endif
Make sure you also add the Android specific package references.
Now, let’s edit our MainPage.xaml.cs
file and the existing event handler there for the button. Let’s modify it to make sure that the device its running on is able to receive push notifications. We’ll also make it get the current FCM registration token. This token is the identifier which Firebase uses to determine which device it should send push notifications to (if you want to send to individual devices). We will be using this later on in this guide.
private async void OnCounterClicked(object sender, EventArgs e)
{
await CrossFirebaseCloudMessaging.Current.CheckIfValidAsync();
var token = await CrossFirebaseCloudMessaging.Current.GetTokenAsync();
await DisplayAlert("FCM token", token, "OK");
}
Configure Cloud Messaging
Now we can start configuring things to make our app receive push notifications. In Firebase this is referred to as (Firebase) Cloud Messaging, or FCM.
First we need to edit the configuration we set up in our MauiProgram.cs
. In the method CreateCrossFirebaseSettings
, add the following:
return new CrossFirebaseSettings(isAuthEnabled: true, isCloudMessagingEnabled: true);
Now we can move on to the platform specific bits. We’ll start with the easy one, namely Android.
Android setup
Follow the Android specific setup in the plugin documentation. Here’s a reference for how your MainActivity.cs
should look like:
If you want a specific icon for your push notification, you can comment out the last line and add an icon to your resources folder.
iOS setup
iOS setup is a bit more tricky. Follow the iOS specific setup in the documentation. This includes a lot of work in the Apple Developer Portal. You’ll need to create an App ID and enable Push Notifications for that App ID. This identifier must match the one set in your Firebase project and ApplicationId
in your MAUI project. Then you’ll need to create a provisioning profile for the newly created App ID. If you’re using a development certificate, make sure to add the physical device your testing on to the provisioning profile.
Follow the iOS specific setup with creating and uploading the APNs authentication key and adding an Entitlements.plist
file to your iOS platform folder.
Hopefully everything should be working now for iOS. Personally I experienced that I wasn’t able to build or debug from my Windows machine so I had to test this from my MacBook Pro on VS for Mac.
Send a test push notification
Finally, we can check if all this work actually… works. The easiest way to do this is from the Firebase Console. Navigate to Cloud Messaging under the Engage menu.
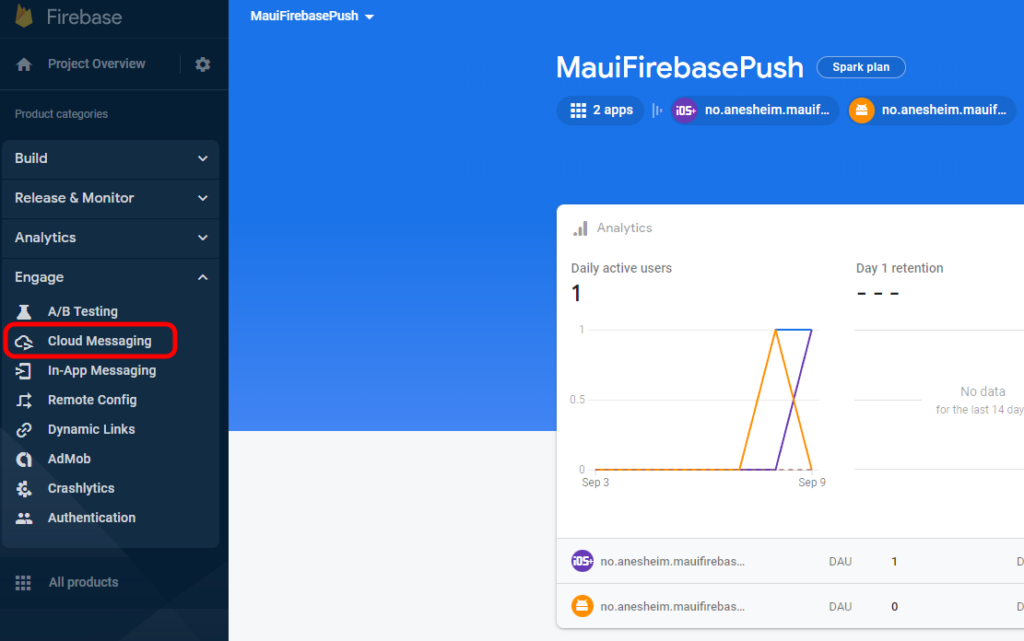
Click “Send your first message” and enter some dummy text as Notification title and Notification text. Then click “Send test message”.
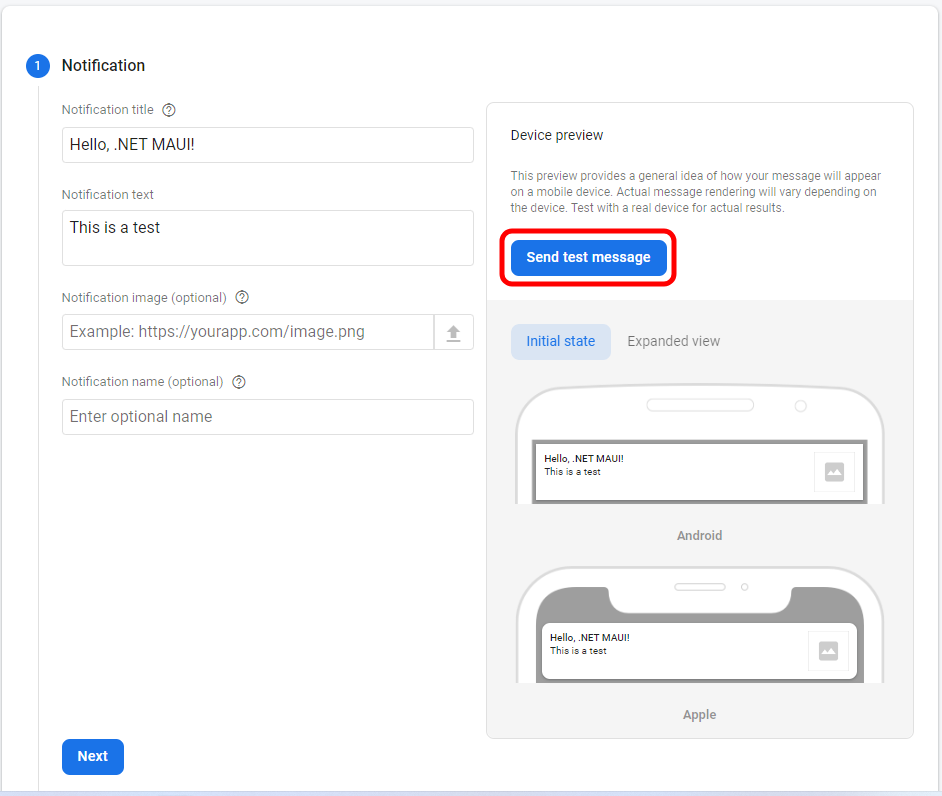
Now you’ll be prompted to enter an FCM registration token. Switch back to Visual Studio and run your app on a physical device. Click the button to receive the FCM registration token for your device.
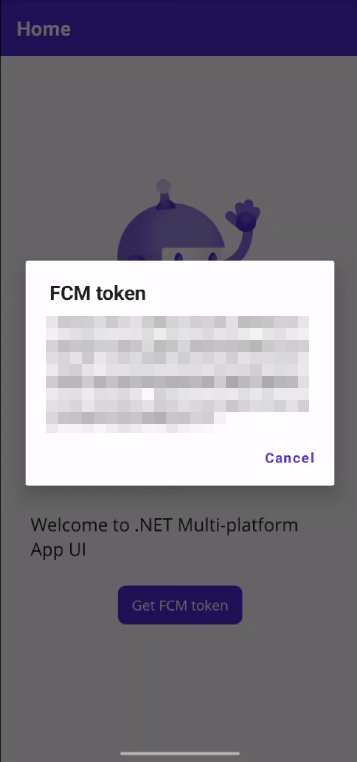
Copy this token by f.ex. setting a breakpoint in your code and copying the value. Go back to the Firebase Console and paste it into the “Test on device” dialog. Click “Test” and you should hopefully receive a push notification now!
Here’s a little test when I do this on my physical iPad device:
Final thoughts
This guide merely scratches the surface of the possibilities around push notifications with Firebase. Normally you would use a backend service to send out messages to your users, either individually or in groups, but for that you can use plenty of Firebase plugins that deals with that side of things. This was meant as a way to show the minimum amount of work you have to do in order to enable recieving push notifications in your client MAUI app. Check out the thorough documentation both for the Firebase plugin mentioned in this post and the official Firebase Cloud Messaging documentation to learn more about how you can leverage this component. You can also check out a sample app that I’ve put up on GitHub.
Why would you need 3rd party libraries to handle tasks as simple as sending notifications? After all, you just need a simple HTTP request.
curl -v –header “apns-topic: ${TOPIC}” –header “apns-push-type: alert” –cert “${CERTIFICATE_FILE_NAME}” –cert-type DER –key “${CERTIFICATE_KEY_FILE_NAME}” –key-type PEM –data ‘{“aps”:{“alert”:”test”}}’ –http2 https://${APNS_HOST_NAME}/3/device/${DEVICE_TOKEN}
where:
CERTIFICATE_FILE_NAME=path to the certificate file
CERTIFICATE_KEY_FILE_NAME=path to the private key file
TOPIC=App ID
DEVICE_TOKEN=device token for your app
APNS_HOST_NAME=api.sandbox.push.apple.com
source: https://developer.apple.com/documentation/usernotifications/sending_push_notifications_using_command-line_tools
That’s one way of doing it! I just wanted to point out that there probably are a lot of plugins out there to do this for you, if you don’t want to create the HTTP requests yourself.
I had more than one query that was not clear to me
1. Should the app be published on the App Store?
2. Is it possible if the app has an add or edit as soon as I add or edit sends a notification to all phones knowing that I’m using firebase realtime (firebasedatabase.net).
3. This is for me personally. I get distracted a lot when I find some articles that direct me to other articles that complement the current article, and this is what happened in this article. Although I visited this article more than once and had difficulty understanding it, I decided to comment on it. Please imagine that I am a beginner in the field of development and list All the steps are complete, the order that will make it easier for me to understand will be as follows explaining all the actions that I will do on firebase and then the steps followed on the application.
Finally, I apologize very much for the length of the comment. If you encounter difficulty, I apologize because I use Google Translator, and that may have made my understanding of the article difficult as well, so I ask you to clarify all the steps because I will follow it step by step and that will make me understand more and it would be excellent if you made a video for the full explanation
Greetings to you
Hi Yousef!
1. If you want your apps to be in the hands of other users, then yes.
2. I’m not too sure what you mean. Do you mean that if you’re editing some realtime Firebase data that a push notification should be sent to your users? I’m pretty sure that would be feasible.
3. I understand that you would like a more thorough article/walkthrough of this. My intention was that I didn’t want to just copy/paste text from other sites so I instead referred to them in this case. I will take this into account though when I am creating articles going forward. Thanks for your feedback!
As for creating videos, my good friend Gerald Versluis has a great YouTube channel where he explains a lot of .NET MAUI stuff. Not sure if he has a video about this yet, but he might some day!
Thank you, Andreas, for sharing great tutorial!
Regarding iOS, here is tutorial describing how to get work FCM for .NET MAUI on iOS platform:https://github.com/michalpr/Docs/blob/main/Firebase_MAUI_iOS_push_notification.md
I followed this and don’t get the push notification event to fire. I get the token however in DidReceiveRegistrationToken. Where am I supposed to get the event to fire? ReceivedRemoteNotification or DidReceiveRemoteNotification
Nevermind, I had missed a couple of points. It worked! Thanks so much! Seriously, couldn’t find this anywhere.
Excellent! Glad it worked out 😊
Thank you! When I build I get the following. Any ideas?
Severity Code Description Project File Line Suppression State
Error MT158 The file ‘C:/Users/jerry/.nuget/packages/xamarin.facebook.gamingserviceskit.ios/12.2.0.1/lib/net6.0-ios15.4/Xamarin.Facebook.GamingServicesKit.resources/FacebookGamingServices.xcframework/ios-arm64_i386_x86_64-simulator/FacebookGamingServices.framework/FacebookGamingServices’ does not exist.
mTeaTimer C:\Program Files\dotnet\packs\Microsoft.iOS.Sdk\16.0.523\targets\Xamarin.Shared.Sdk.targets 663
Hi Jerry! I tried building against my Mac with XCode 14 and didn’t get any errors. You could try filing an issue under the GitHub repository for the package used.
I am also facing the same error when build on iOS.
The file ‘C:/Users/abcd/.nuget/packages/xamarin.facebook.gamingserviceskit.ios/12.2.0.1/lib/net6.0-ios15.4/Xamarin.Facebook.GamingServicesKit.resources/FacebookGamingServices.xcframework/ios-arm64_i386_x86_64-simulator/FacebookGamingServices.framework/FacebookGamingServices’ does not exist.
I have followed each steps below but nothing worked.
https://github.com/michalpr/Docs/blob/main/Firebase_MAUI_iOS_push_notification.md
https://github.com/TobiasBuchholz/Plugin.Firebase/blob/development/docs/auth.md
Could you let us know any workaround?
Is this is causing because of long path of package?
Hi, i got the same error, do you fixed it? Thanks
Has anyone solved this yet?
Hi Andreas,
Thank you for the detailed guide for implementing push notifications in a Maui application. I did everything according the guidelines and it’s all working fine. However, what do I need to implement in this in order to make this also work on the android emulator in Maui?
Thank you in advance.
Hi, Brian! Glad I could help! I don’t think that the Android emulator has support for receiving push notifications, at least not when I’ve tested. I think you’re stuck with having to test it on a physical device. Not sure about iOS though, but I think it’s the same story there.
How do i navigate to different pages after recieving Notification.
I haven’t tried this myself in .NET MAUI, but I recommend that you read the documentation for the plugin used in this post. The sample app could also maybe give some pointers on how to do this: https://github.com/TobiasBuchholz/Plugin.Firebase/blob/development/docs/cloud_messaging.md
Thank you.
This work for me
CrossFirebaseCloudMessaging.Current.NotificationTapped += Current_NotificationTapped;
Can you please give a sample on NotificationTapped event in iOS? This works in android but not in iOS? I badly need it. Thanks in advance.
Larie
Hi
Thanks for the article. Would this work on .Net 7 MAUI too?
Thanks
Quinton
I’m pretty sure it will yes, as long as the Nuget package supports it
I ask you, my friend, to write a topic that explains how to send a notification to a specific device. Because I’m having trouble doing that and it always returns the unauthorized request
My friend, I tried to implement this with .net 7, and it didn’t work. Can you explain to solve this
Thanks Andres. This looks useful.
The problem is that I do not get FCM Token
I am trying to get it just when clicking a button. Does not work in emulator, iOS and does not work in Android device. I tried to debug, but FCM token is always null.
https://github.com/TobiasBuchholz/Plugin.Firebase/blob/c2d5c5f8bfb598c4cb8439a0ac2f6db6cee92c69/src/Android/CloudMessaging/FirebaseCloudMessagingImplementation.cs#L121
Exception details:
Couldn’t retrieve FCM token
at Plugin.Firebase.CloudMessaging.FirebaseCloudMessagingImplementation.GetTokenAsync()
at MobileApp.Pages.Feedback.SendFeedback()
at Microsoft.AspNetCore.Components.ComponentBase.CallStateHasChangedOnAsyncCompletion(Task task)
at Radzen.Blazor.RadzenButton.OnClick(MouseEventArgs args)
at Microsoft.AspNetCore.Components.ComponentBase.CallStateHasChangedOnAsyncCompletion(Task task)
at Microsoft.AspNetCore.Components.RenderTree.Renderer.GetErrorHandledTask(Task taskToHandle, ComponentState owningComponentState)
Yo. Thansk for the guide. I got it to work.
Do you know what I need to do if I want to receive the notification when the app isn’t running. Like in the background?
If you know a guide to it or something I would be very thankfull 🙂
ciao, I tried to use it for android only… i take your sample code from GitHub, I imported my google-service.json file and set it as googleServiceJson, run the application on physical device an copy the FCM token, I create campaign message for my app and my token and I run it. Result : No messages/notification arrived on my physical device. is there something wrong? hits are very appreciate.
Thanks for the post, I have two questions, 1) in .net 7.0 the option events.AddAndroid() is not available, 2) the same code works for a .net Maui Hibryd application.
Push notification not working when app is not running, It should work like other applications e.g. YouTube app we get notification even app is not running.